本篇主要介绍如何在ASP.NET Core MVC应用中基于MySQL使用EF 。在阅读本篇文章之前,应该有一定的ASP.NET Core基础。
系统环境
1、.NET Core SDK 2.2;
2、Visual Studio 2017 或 2019。
参考
1、教程:在 ASP.NET MVC Web 应用中使用 EF Core 入门
2、Applying Seed Data To The Database
主体
一、创建WEB项目
打开 Visual Studio,选择 “ASP.NET Core Web 应用程序”,创建一个新项目。
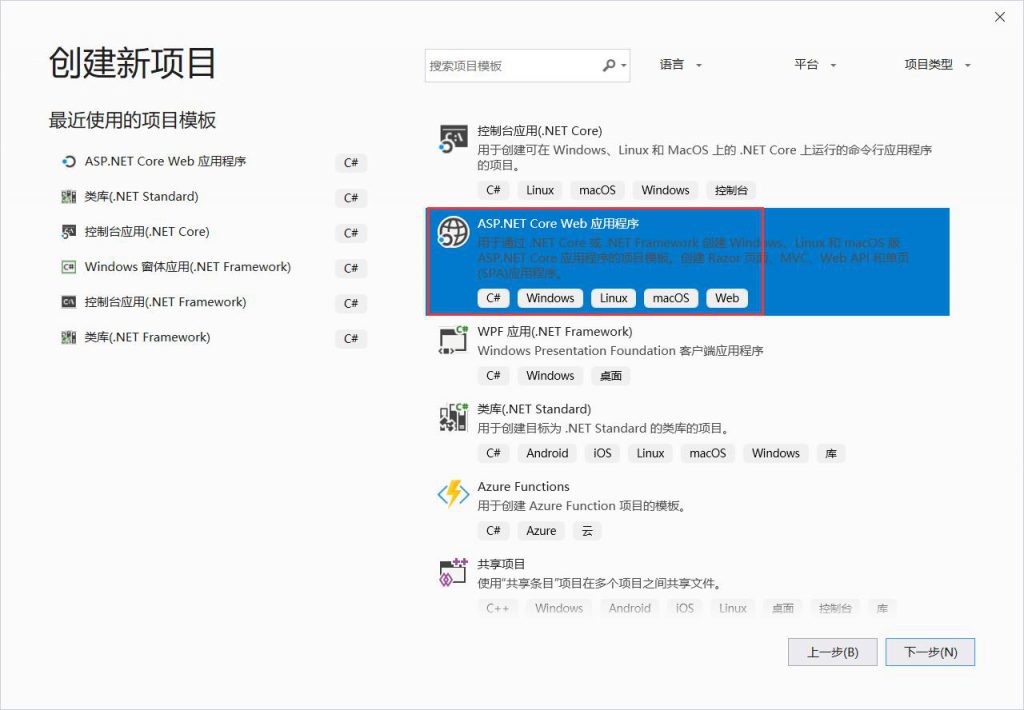
选择“.NET Core”、“ASP.NET Core 2.2”和“Web 应用程序(模型-视图-控制器)”模板。
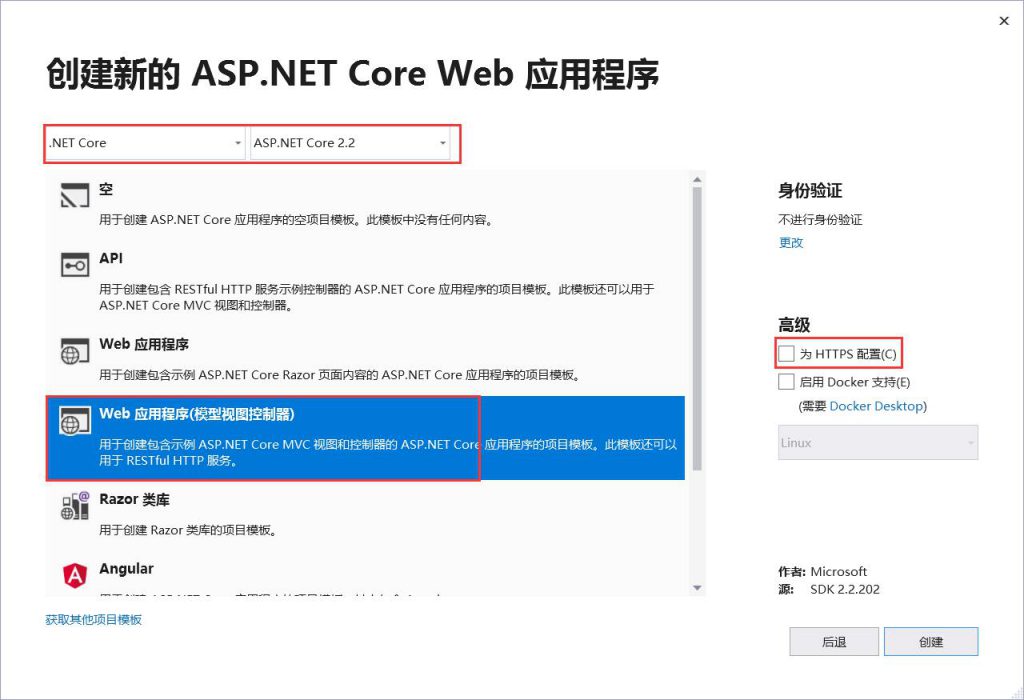
二、添加Nuget包
在进行代码编写之前,首先添加EF和Mysql依赖的数据库驱动组件。在NuGet管理器中为项目添加以下三个包。
- Microsoft.EntityFrameworkCore.Tools
- MySql.Data
- MySql.Data.EntityFrameworkCore
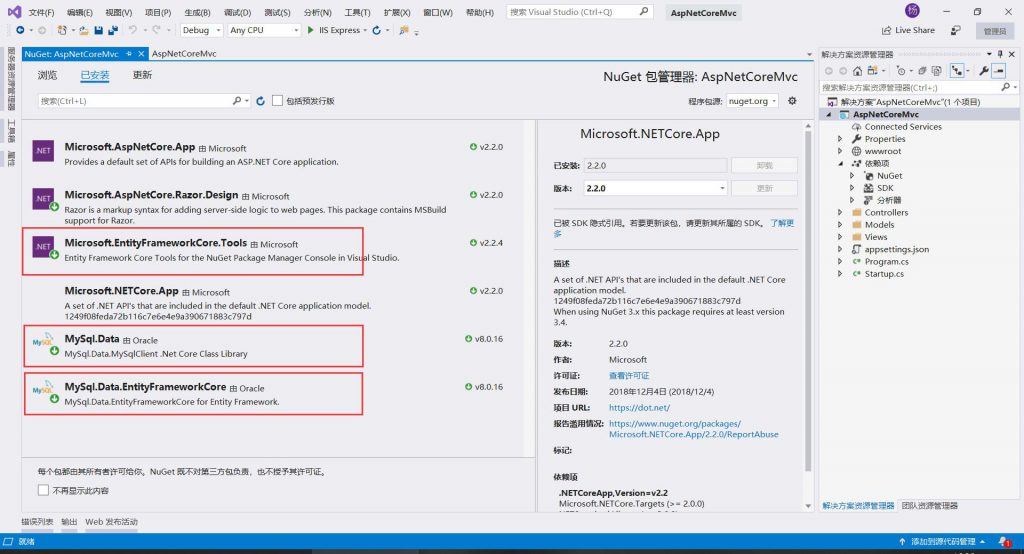
三、配置数据库连接字符串
在appsettings.json中添加节点,代码如下:
"ConnectionStrings": {
"DevelopConnectString": "Data Source=localhost;port=3306;Initial Catalog=test;uid=root;password=123456;Character Set=utf8;sslmode=none"
},
四、编写DbContent
1、在Models文件夹下面创建文件夹Entity,并在Entity文件夹下创建实体Students。代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Threading.Tasks;
namespace AspNetCoreMvc.Models.Entity
{
public class Students
{
[Key]
public int Id { get; set; }
[MaxLength(60)]
public string Name { get; set; }
[MaxLength(2)]
public string Sex { get; set; }
[MaxLength(30)]
public string Phone { get; set; }
}
}
2、在Models文件夹中添加CoreDbContent,代码如下:
using AspNetCoreMvc.Models.Entity;
using Microsoft.EntityFrameworkCore;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace AspNetCoreMvc.Models
{
public class CoreDbContent : DbContext
{
public CoreDbContent(DbContextOptions<CoreDbContent> options) : base(options)
{
}
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
}
public DbSet<Students> Students { get; set; }
}
}
3、在Startup文件中的ConfigureServices方法中添加以下代码,用于注册数据库服务。
//配置数据库实体依赖注入
services.AddDbContext<CoreDbContent>(options => options.UseMySQL(Configuration.GetConnectionString("DevelopConnectString")));
此时 ConfigureServices方法 如下所示:
public void ConfigureServices(IServiceCollection services)
{
services.Configure<CookiePolicyOptions>(options =>
{
// This lambda determines whether user consent for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
//配置数据库实体依赖注入
services.AddDbContext<CoreDbContent>(options => options.UseMySQL(Configuration.GetConnectionString("DevelopConnectString")));
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_2);
}
到此为止,如果不希望在数据库初始化后添加默认数据,可以在程序包管理控制台中执行以下两个命令初始化数据库。
如果希望在创建数据库时添加默认数据,那么可以跳过这一步。
Add-Migration Init
Update-DataBase
4、添加初始化数据
在Models文件下添加类DbDataInitializer,并编写如下代码:
using AspNetCoreMvc.Models.Entity;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace AspNetCoreMvc.Models
{
public class DbDataInitializer
{
public static void Initialize(CoreDbContent context)
{
//如果没有数据库,那么新建数据库
context.Database.EnsureCreated();
if (context.Students.Count() > 0)
{
return;
}
Students[] stus = new Students[]{
new Students()
{
Name = "小明",
Sex = "男",
Phone = "123456"
},
new Students()
{
Name = "小红",
Sex = "女",
Phone = "456789"
}
};
context.Students.AddRange(stus);
if (context.SaveChanges() == 0)
{
throw new Exception("写入默认数据失败。");
}
}
}
}
修改Program.cs中的Main方法中的代码为以下代码。
public static void Main(string[] args)
{
var host = CreateWebHostBuilder(args).Build();
using (var scope = host.Services.CreateScope())
{
var services = scope.ServiceProvider;
try
{
var context = services.GetRequiredService<CoreDbContent>();
DbDataInitializer.Initialize(context);
}
catch (Exception ex)
{
var logger = services.GetRequiredService<ILogger<Program>>();
logger.LogError(ex, "An error occurred while seeding the database.");
}
}
host.Run();
}
5、编写测试代码
修改HomeController为以下代码:
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using AspNetCoreMvc.Models;
using AspNetCoreMvc.Models.Entity;
namespace AspNetCoreMvc.Controllers
{
public class HomeController : Controller
{
private readonly CoreDbContent _db;
public HomeController(CoreDbContent content)
{
_db = content;
}
public IActionResult Index()
{
try
{
using (_db)
{
List<Students> students = _db.Students.ToList();
if(students!=null && students.Count > 0)
{
ViewBag.Students = students;
}
else
{
ViewBag.Students = new List<Students>();
}
return View();
}
}
catch(Exception ex)
{
throw new Exception($"获取学生数据==信息失败,{ex.Message}");
}
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
在HomeController中主要修改的有:
- 添加构造函数,获取依赖注入的CoreDbContent
- 修改Index方法
6、运行测试
在浏览器中打开本地测试页面后,会将添加的测试数据打印出来。如果未添加测试数据,将显示空白。
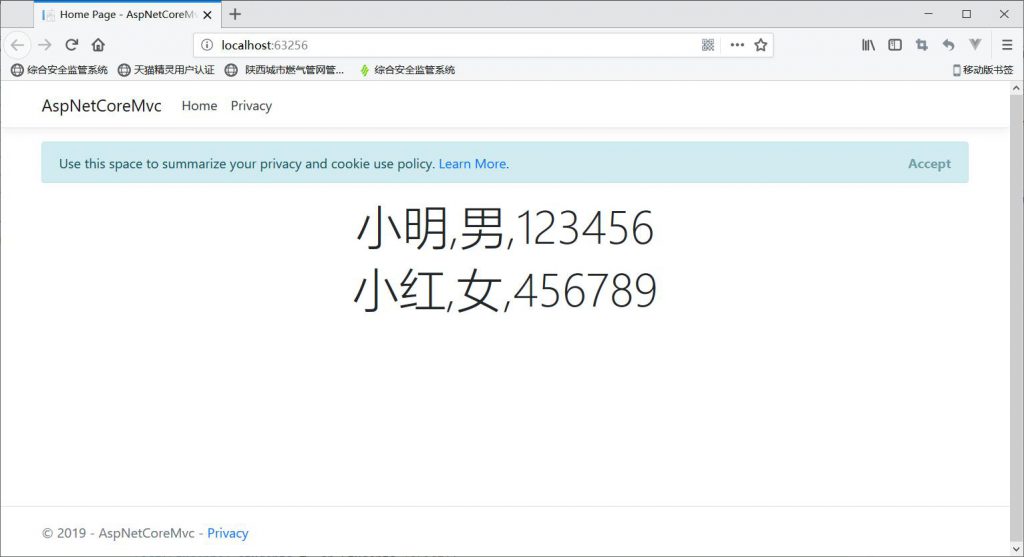
7、Demo下载
文章评论